How to Create a Chat App in Android
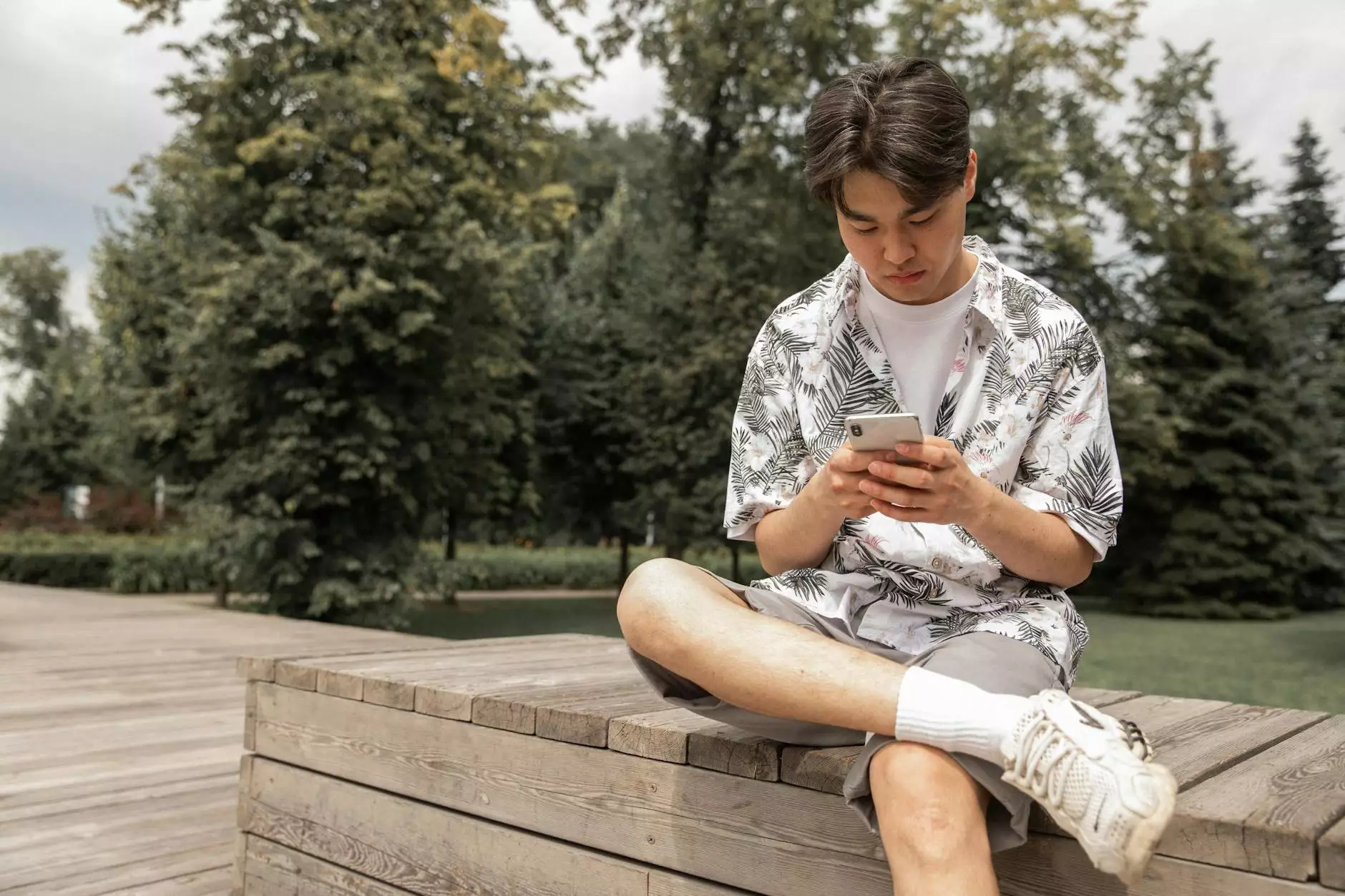
In today's digital age, messaging applications have become an integral part of everyday communication. Whether for personal use or business networking, the demand for custom chat applications is on the rise. This article will guide you through the process of how to create a chat app in Android, outlining the essential tools, technologies, and best practices you will need to succeed.
Understanding the Basics of Chat Applications
A chat app allows users to send text messages, images, audio files, and more, in real-time. The core functionalities typically include:
- User Registration: Users can create accounts and log in.
- Chat Functionality: Sending and receiving messages.
- Media Sharing: Users can share images, videos, and documents.
- Push Notifications: Users are notified of new messages even when the app is not in use.
- Group Chats: Users can create group conversations.
Choosing the Right Tools and Technologies
To develop a successful chat app, you will need to select appropriate technologies. Here’s a look at some of the essential components:
- Programming Language: Android applications are primarily built using Java or Kotlin, with Kotlin gaining popularity for its modern features and streamlined syntax.
- Backend Server: To manage communication and data storage, you’ll need a backend. Common choices include Node.js, Django, or Firebase.
- Database: You'll store user data and chat histories in a database like Firebase Firestore, SQLite, or MySQL.
- Real-Time Communication Technology: For real-time messaging, consider using WebSocket-based solutions or Firebase Realtime Database.
- UI/UX Framework: Leverage Android’s Material Design components to create appealing user interfaces.
Step-by-Step Guide to Creating a Chat App in Android
Now that we have laid the groundwork, let’s go through the step-by-step process of how to create a chat app in Android.
Step 1: Set Up Your Development Environment
Before you start coding, ensure you have the necessary tools installed:
- Android Studio: The official IDE for Android development, which provides all the essential tools.
- Java Development Kit (JDK): Necessary for Java-based programming.
- Emulator or Physical Device: To test your application in real-time.
Step 2: Create a New Android Project
Open Android Studio and create a new project:
- Select “Start a new Android Studio project.”
- Choose a project template (e.g., “Empty Activity”).
- Name your project and set your preferred programming language.
Click “Finish” to create your project.
Step 3: Implement User Registration
The next step is to implement user registration. You can utilize Firebase Authentication for simplicity. Here’s a brief outline:
- Add Firebase to your project through the Firebase Console.
- Include necessary dependencies such as Firebase Authentication in your \texttt{build.gradle} file.
- Design your registration screen using XML layouts.
- Create a registration method that handles user inputs and communicates with Firebase Authentication.
Step 4: Build the Chat Interface
Designing the chat layout is crucial for user experience. Use a RecyclerView to display chat messages:
RecyclerView recyclerView = findViewById(R.id.recycler_view); // Set up the layout manager and adapter.Define an adapter class to manage message items, enabling real-time updates with LiveData or similar technologies.
Step 5: Implement Real-Time Messaging
To allow users to send and receive messages in real-time, you’ll implement a backend service. Using Firebase Realtime Database, here’s how:
- Store messages in a database node dedicated to chat.
- Utilize listeners to update the chat interface as messages are sent or received.
- Ensure you handle edge cases, such as connection loss.
Step 6: Add Media Support
To enhance your chat app, you can allow users to share images or files. You can use built-in Android libraries to access the device’s storage and incorporate the following:
- Implement Intent to select images from the gallery.
- Upload the image to Firebase Storage using the Firebase SDK.
- Save the file URL in the Firebase Realtime Database alongside the message data.
Step 7: Implement Group Chat Functionality
To create group chats, follow these steps:
- Allow users to create a chat group and add members.
- Persist group chat data in the database.
- Modify your messaging listener to handle messages sent to groups.
Testing Your Chat Application
After implementing all the features, it's crucial to thoroughly test your application:
- Perform unit tests to ensure individual components function correctly.
- Conduct UI tests to verify the user interface is responsive.
- Test real-time features under various network conditions.
Publishing Your Chat App
Once your app is tested and ready, it’s time to publish:
- Prepare your app for release by configuring the build settings.
- Generate a signed APK or App Bundle.
- Publish your app to the Google Play Store following their guidelines.
Best Practices for Chat App Development
Here are some additional best practices to keep in mind when creating a chat app:
- Security: Ensure user data is encrypted and authenticated, especially for sensitive information.
- Scalability: Design your app to handle large user bases without performance issues.
- User Experience: Prioritize a clean UI, responsive design, and quick load times.
- Feedback Loop: Incorporate user feedback to iterate and improve your application continuously.
Conclusion
Creating a chat app in Android is an exciting journey that involves various skills and technologies. By following the steps outlined in this article, you can build a robust and feature-rich messaging application tailored to your users' needs. Remember, the key to success lies in understanding your audience, implementing best practices, and continuously improving your app based on feedback.
With dedication and the right resources, you will master how to create a chat app in Android and position your application to stand out in the crowded marketplace. For further resources and assistance, consider reaching out to platforms like nandbox.com to explore mobile development solutions and software services that can support your journey.